Eğitimler
StringAppendOperator
String'leri StringAdditionOperator kullanarak diğer veri nesneleriyle birleştirebildiğiniz gibi, +=
operatörünü ve concat()
yöntemini kullanarak Dizelere şeyler ekleyebilirsiniz. +=
İşleci ve concat()
yöntemi aynı şekilde çalışır, bu yalnızca hangi stili tercih ettiğinizle ilgilidir. Aşağıdaki iki örnek her ikisini de gösterir ve aynı Dizeyle sonuçlanır:
String stringOne = "A long integer: "; // using += to add a long variable to a string: stringOne += 123456789;
veya
String stringTwo = "A long integer: "; // using concat() to add a long variable to a string: stringTwo.concat(123456789);
Her iki durumda da, stringOne
eşittir "Uzun bir tamsayı: 123456789". +
İşleci gibi, bu işleçler de veri nesnelerinin birleşiminden daha uzun dizeler oluşturmak için kullanışlıdır.
Gerekli Donanım
- Arduino veya Genuino Kurulu
Devre
Bu örnek için devre yoktur, ancak kartınızın bilgisayarınıza USB ile bağlanması ve Arduino Yazılımının (IDE) seri monitör penceresinin açık olması gerekir.
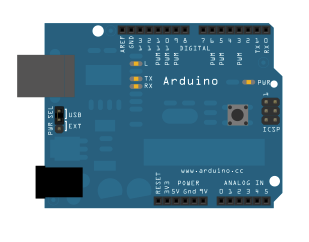
Kod
/*
Appending to Strings using the += operator and concat()
Examples of how to append different data types to Strings
created 27 Jul 2010
modified 2 Apr 2012
by Tom Igoe
This example code is in the public domain.
http://www.arduino.cc/en/Tutorial/StringAppendOperator
*/
String stringOne, stringTwo;
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
stringOne = String("Sensor ");
stringTwo = String("value");
// send an intro:
Serial.println("\n\nAppending to a String:");
Serial.println();
}
void loop() {
Serial.println(stringOne); // prints "Sensor "
// adding a string to a String:
stringOne += stringTwo;
Serial.println(stringOne); // prints "Sensor value"
// adding a constant string to a String:
stringOne += " for input ";
Serial.println(stringOne); // prints "Sensor value for input"
// adding a constant character to a String:
stringOne += 'A';
Serial.println(stringOne); // prints "Sensor value for input A"
// adding a constant integer to a String:
stringOne += 0;
Serial.println(stringOne); // prints "Sensor value for input A0"
// adding a constant string to a String:
stringOne += ": ";
Serial.println(stringOne); // prints "Sensor value for input"
// adding a variable integer to a String:
stringOne += analogRead(A0);
Serial.println(stringOne); // prints "Sensor value for input A0: 456" or whatever analogRead(A0) is
Serial.println("\n\nchanging the Strings' values");
stringOne = "A long integer: ";
stringTwo = "The millis(): ";
// adding a constant long integer to a String:
stringOne += 123456789;
Serial.println(stringOne); // prints "A long integer: 123456789"
// using concat() to add a long variable to a String:
stringTwo.concat(millis());
Serial.println(stringTwo); // prints "The millis(): 43534" or whatever the value of the millis() is
// do nothing while true:
while (true);
}
See Also
- String object- String nesneleri için Referansınız
- CharacterAnalysis- Karşılaştığımız karakter türünü tanımamıza izin veren operatörleri kullanıyoruz.
- StringAdditionOperator- Çeşitli yollarla birlikte dizeler ekleyin.
- StringCaseChanges- Bir dizenin durumunu değiştirin.
- StringCharacters- Bir dizede belirli bir karakterin değerini alır / ayarlar.
- StringComparisonOperators- Bir dizede belirli bir karakterin değerini alır / ayarlar.
- StringConstructors- Dize nesnelerini başlatın.
- StringIndexOf- Dizede bir karakterin ilk / son örneğini arayın.
- StringLength- Bir dizginin uzunluğunu al.
- StringLengthTrim- Bir dizenin uzunluğunu alın ve kesin.
- StringReplace- Bir dizedeki karakterleri tek tek değiştirin.
- StringStartsWithEndsWith- Belirli bir dizenin hangi karakterlerle / alt dizelerle başladığını veya bittiğini kontrol edin.
- StringSubstring- Belirli bir dize içinde "ifadeler" arayın.
- StringToInt- Bir String'i tamsayıya dönüştürmenizi sağlar.